Date Picker in Django Frontend
I am going to show you how to simply implement the date picker in Django using jQuery. It’s amazingly simple and straight forward.
django-admin startproject djangodatepicker
python manage.py startapp datepicker
from django.db import models
# Create your models here.
class Datepicker(models.Model):
name = models.CharField(max_length=1000)
dob = models.DateField(null=True, blank=True)
class Meta:
verbose_name = 'Date Picker'
ordering = ('name',)
def __str__(self):
return '{}'.format(self.name)
from django.forms import ModelForm
from django import forms
from .models import Datepicker
class DatepickerForm(ModelForm):
name = forms.CharField(required=False)
dob = forms.DateField(required=False)
class Meta:
model = Datepicker
fields = ['name', 'dob']
from django.views.generic import CreateView
from .forms import DatepickerForm
from django.urls import reverse_lazy
from .models import Datepicker
# Create your views here.
class DatePickerView(CreateView):
model = Datepicker
form_class = DatepickerForm
template_name = 'datepicker/datepicker.html'
success_url = reverse_lazy('datepicker:dates')
from django.urls import path
from datepicker import views
app_name = 'datepicker'
urlpatterns = [
path('dates/', views.DatePickerView.as_view(), name='dates'),
]
urlpatterns = [
path('datepicker/', include('datepicker.urls', namespace='datepicker'))
]
Make sure to load the jQuery library before loading the jQuery UI library. The libraries must be loaded only once on the page, otherwise the error is thrown.
{% load static i18n %}<!DOCTYPE html>
{% get_current_language as LANGUAGE_CODE %}
<html lang="{{ LANGUAGE_CODE }}">
<head>
<meta charset="utf-8">
<!-- Start the jquery library used to load date picker in django project -->
<!-- ✅ Load CSS file for jQuery ui -->
<link
href="https://code.jquery.com/ui/1.12.1/themes/ui-lightness/jquery-ui.css"
rel="stylesheet"/>
<!-- ✅ load jQuery ✅ -->
<script
src="https://code.jquery.com/jquery-3.6.0.min.js"
integrity="sha256-/xUj+3OJU5yExlq6GSYGSHk7tPXikynS7ogEvDej/m4="
crossorigin="anonymous"></script>
<!-- ✅ load jquery UI ✅ -->
<script
src="https://cdnjs.cloudflare.com/ajax/libs/jqueryui/1.12.1/jquery-ui.min.js"
integrity="sha512-uto9mlQzrs59VwILcLiRYeLKPPbS/bT71da/OEBYEwcdNUk8jYIy+D176RYoop1Da+f9mvkYrmj5MCLZWEtQuA=="
crossorigin="anonymous"
referrerpolicy="no-referrer"></script>
<!-- End the jquery library used to load date picker in django project -->
</head>
<body>
<div class="container-fluid">
{% if messages %}
{% for message in messages %}
<div id="alert" class="alert alert-dismissible {% if message.tags %}alert-{{ message.tags }}{% endif %}">
{{ message }}
</div>
{% endfor %}
{% endif %}
{% block content %}
<p>Use this document as a way to quick start any new project.</p>
{% endblock content %}
</div>
<!-- /.container-fluid -->
</div>
<!-- End of Main Content -->
</body>
</html>
{% extends "base.html" %}
{% load i18n %}
{% block title %}
Date Example
{% endblock title %}
{% block content %}
<form method="post" action="" novalidate>
{% csrf_token %}
{{ form.as_p }}
<button> Submit </button>
</form>
<script type="text/javascript">
$(function() {
$('#id_dob').datepicker( {
changeMonth: true,
changeYear: true,
showButtonPanel: true,
yearRange: "2022:2099",
dateFormat: 'mm/dd/yy'
});
});
</script>
{% endblock %}
The image below shows the date picker showing up when you click on the Dob field. Thats all!
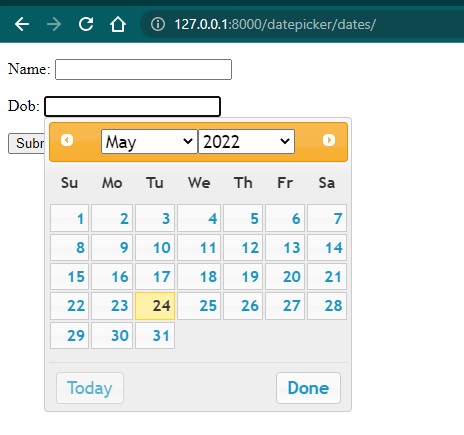
Solve $(…).datepicker is not a function #
The “$(…).datepicker is not a function” jQuery error occurs for multiple reasons:
- Forgetting to include the jQuery UI library
- Loading the jQuery UI library before the jQuery library
- Loading the jQuery library twice
- Specifying an incorrect path to the jQuery files.
- Using a custom jQuery UI library that does not have a datepicker
To solve the “$(…).datepicker is not a function” jQuery error, make sure to load the jQuery library before loading the jQuery UI library. The libraries have to be loaded only once on the page, otherwise the error is thrown.
You can get full code on GitHub link. Leave your comments below if you need to say anything.